Project Title: FomoFoto
Overview
FomoFoto is a robust yet simple image-editing tool. Users interact with the application through worded commands from their keyboard and receive visual feedback from it through the displayed image on the application.
Unlike other heavy image editors, FomoFoto has a gentle learning curve, removing clutter while still retaining essential features. All features and their implementations are well documented in guides for both users and developers respectively.
Summary of contributions
Major Enhancements:
-
Importing of images.
-
What it does: Allow users to import images into FomoFoto for editing. Imported images are added to an Album in FomoFoto that can be opened for edits such as Image Transformation and Filters.
-
Justification: This feature is core to the operation of FomoFoto. Without the ability to import images, an image editor cannot function properly since there is no image to modify.
-
Highlights: This enhancement is tough to implement as it requires many checks to ensure the image is valid. Images which do not fit the rule set such as wrong name formats are rejected. Users are also given the flexibility to import a single image or a directory.
-
-
Overall user interaction and interface/experience design.
-
What it does: FomoFoto has an intuitive, human-centric design. Users are able to see the images they are editing, the information of images in the Album, their details and all edits applied.
-
Justification: A good user interface facilitates easy usage of the editor. Users are able to get a good overview of the edits they are doing simply by observing the image and information available on the screen. This feature comprises of five components:
-
Initial Panel
- Contains a quick start message on FomoFoto’s first start, giving users a high-level overview of the commands available. -
Image Panel
- Displays the image currently opened for editing. -
Album Images Panel
- Displays all images imported to FomoFoto’s Album. -
EXIF Profile Panel
- Displays core details such as Time and Location Taken about the opened image shown on the Image Panel. -
Command History Panel
- Displays all previously applied edits on the opened image shown on the Image Panel.
-
-
Highlights: This enhancement is core to the operation of FomoFoto. Since we are dealing with images, it is paramount to show the item that is being worked on. Without it, there is no visual cue if the edits being carried out is accurate. Careful attention has been paid to ensure images are aligned accurately with a clean colour scheme.
-
Minor Enhancements:
-
Tab Command.
-
Added a Tab Command that allows the user to interact with the Information Panel without using a mouse.
-
-
Inclusion of
sample
image folder.-
Added a folder containing a list of valid and invalid images. These images can be used for command and feature testing.
-
Example of sample images:
-
validJPGTest.jpg
-
invalidPDFTest.pdf
-
-
-
Image and Album classes.
-
Breaks down imported images in FomoFoto to an extensible Image class which is added to an Album. This ensures all images are valid and allows the creation of other commands by tapping on Album’s methods.
-
Other Contributions
Documentation:
-
Addendum tweaks to existing contents of the User Guide: #236
Community:
-
Core PRs Contributed:
#10, #56, #75, #87, #105, #111, #119, #125, #156, #163, #193, #195, #202, #203, #215, #239, #246 -
Core Issues Opened:
#11, #13, #30, #31, #41, #42, #43, #44, #73, #76, #77, #78, #79, #81, #82, #83, #85, #95, #109, #149 -
Core Issues Reviewed:
#64, #108, #148 #165, #166, #169, #170, #175, #176, #177, #178, #181, #183, #185, #186, #236
Tools:
-
Integrated TravisCI to the team repo.
-
Integrated Coveralls to the team repo.
-
Added Issue Templates to the team repo.
Contributions to the User Guide
The section below showcases my contributions towards the User Guide. |
Quick Start
System Requirements:
-
Windows 10 or newer / OS X 10.8 or newer.
-
Java 9 or newer.
Follow the steps below to set up your computer to run FomoFoto:
-
Ensure your system meets the system requirements.
-
Download the latest FomoFoto.jar here.
-
Copy the JAR to the folder you want to use as the home folder for your FomoFoto application.
-
Double-click the JAR to start the application. The Graphical User Interface should appear in a few seconds.
Figure 1. Home Page of FomoFoto -
You have just successfully setup FomoFoto on your device. To see the command available in FomoFoto, refer to [Features] for details of each command. A step-by-step walkthrough of FomoFoto is available in the next section: [A Walk Through].
Import an Image : import
Imports images to FomoFoto’s Album from a specified file path or a folder.
Format: import FILEPATH
Example of single file path import:
-
import C:\Users\Addison\Desktop\sample.jpg
-
import C:\Home\sample.png
Example of folder import:
-
import C:\Users\Addison\Desktop
-
import C:\Home
For folder import, invalid files are skipped and only valid images will be imported.
Images should be of the following formats: bmp , jpg , jpeg , png , tif , tiff or gif . Images with tif or tiff formats are not rendered on screen due to a bug with Java but edits made will still be applied.
|
Additionally, images must also adhere to the following additional requirements:
-
Not be hidden or prepended by a
.
dot identifier. -
Not be over 10MB in size.
-
Not have the same name as an existing image in the Album.
Tab Switching: tab
Cycle between available tabs in the side Information Panel.
Format: tab
The three available tabs are listed below:
-
Album Images
-
Displays all images currently opened in the Album and available for editing.
-
-
EXIF Profile
-
Displays all ancillary tags attached to the currently opened image such as metadata information like Date and Time and Copyright information.
-
-
Command History
-
Display all Image Transformation and Image Filters applied to the currently opened image.
-
Contributions to the Developer Guide
The section below showcases my contributions towards the Developer Guide. |
Album and Image Class
The Album
class is also implemented using the Singleton pattern. This design choice was purposely made as only a single instance of Album
should reside in FomoFoto at any one time. This design also reduces the need for passing instance variables across all logic and model components.
The core functions of the Album
class are listed below:
-
Stores a reference to all imported images as List.
-
Contains various helper methods to access the
assets
directory, the storage for the raw image files. -
Fires property changes to listeners attached to the
Notifier
to redraw UI elements.
The Model also comprises of a smaller subclass, Image
.
The Image
class encapsulates the fields of a raw image file. This design choice allows for better abstraction between classes and easy reuse between commands.
The core functions of the Image
class are listed below.
-
Validation checks to see if a raw image is a valid Image.
-
Keeps a command history of edits executed on this Image.
UI component
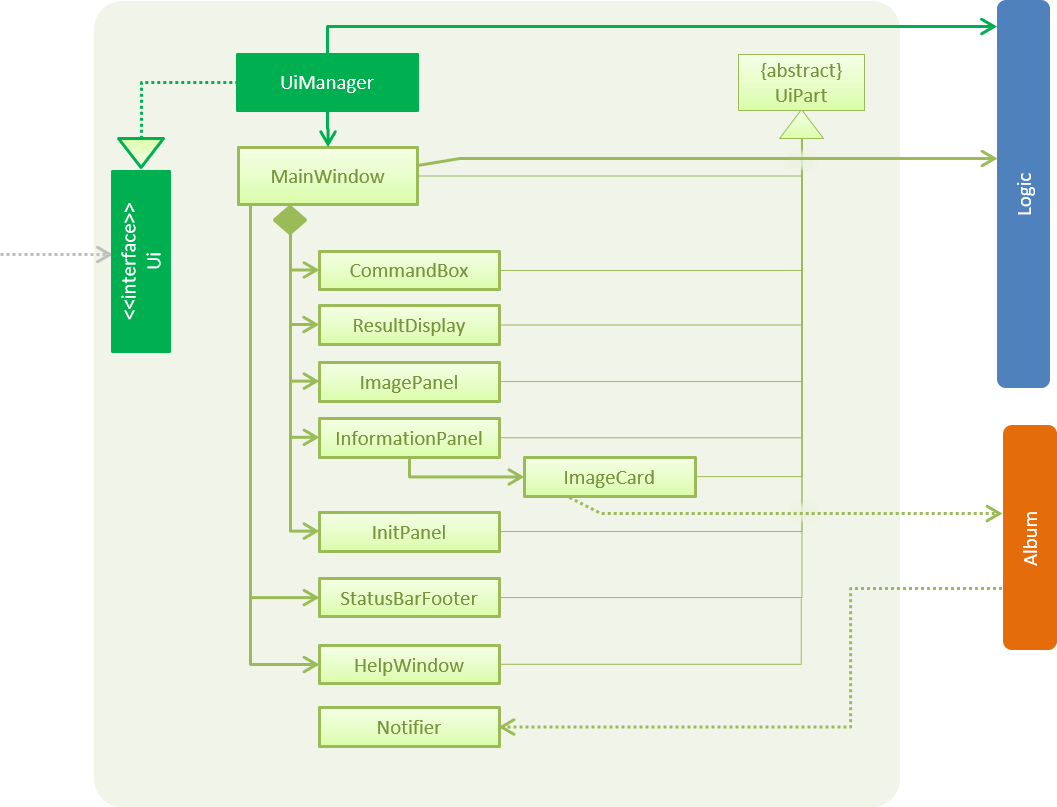
API : Ui.java
The UI consists of a MainWindow
that is made up of the following
components:
-
CommandBox
-
ResultDisplay
-
ImagePanel
-
InitPanel
-
InformationPanel
-
StatusBarFooter
-
HelpWindow
All components, including the MainWindow
, inherit from the abstract UiPart
class.
The InformationPanel
comprises of three tabs:
-
Album Images
-
Displays all images currently opened in the
assets
directory and available for editing.
-
-
EXIF Profile
-
Displays all ancillary tags attached to the currently opened image such as metadata information like Date and Time and Copyright information.
-
-
Command History
-
Display all Image Transformation and Image Filters applied to the currently opened image.
-
The UI
component is supplemented by a Notifier
class. The class implements Java’s propertyChangeListener
as a means of drawing updates to the User Interface.
For example, the ImageView
component draws updates to an opened image in the following sequence:
-
Register the
ImageView
component with theNotifier
class. -
Notifier
is called whenOpen
command is executed with a given Property Name. -
Notifier
fires an alert with the Property Name to all registered listener. -
ImageView
checks if the Property Name is pertaining to it and redraws the image shown by accessingAlbum
.
The UI component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml files that are in the src/main/resources/view directory.
|
Import feature
The Import Command allows users to import a file of an image format into FomoFoto for editing.
Current implementation
The implementation of the import feature allows for users to either:
-
Import a single image file.
-
Import all image files in a directory.
-
Import a sample list of image files.
Image file must adhere to a subset of the following formats as describe by its MIME type standardized in IETF’s RFC 6838:
-
.bmp
-
.jpeg
or.jpg
-
.png
-
.tif
or.tiff
-
.gif
Images with .tif or .tiff formats are not rendered on screen due to a limitation with JavaFX but edits made will still be applied.
|
Images must also adhere to the following additional requirements:
-
Not be hidden or prepended by a
.
dot identifier. -
Not be over 10MB in size.
-
Not have the same name as an existing image in the Album.
Implementation of these checks can be found in ImportCommandParser .
|
Images found in the given arguments will be copied to an assets
directory created at runtime in FomoFoto’s home directory. This is facilitated by the Album
class and ImportCommandParser
and contains the following operation:
-
Album#refreshAlbum()
- Fires a property change to all registered listeners. Listeners addressing this particular property will trigger and update accordingly. -
ImportCommandParser#parse(String)
- Takes in an absolute path, perform sanity checks on availability, size, format and copies the image toassets
directory.
Additionally, the import sample
command is supported by the ResourceWalker
class. This class traverses through a sample directory of valid image files and populates FomoFoto.
Example usage scenario:
-
The user launches the application and enters
import C:\Users\Addison\Desktop\sample.png
-
ImportCommandParser
takes in arguments and performs validation on a given path. Image is copied toassets
directory and added to Album if it is valid. -
Album
display the imported image to UI by calling theNotifier
class which calls on the display panel listening to the notifier. -
InformationPanel
updates to reflect the imported image in the Album.
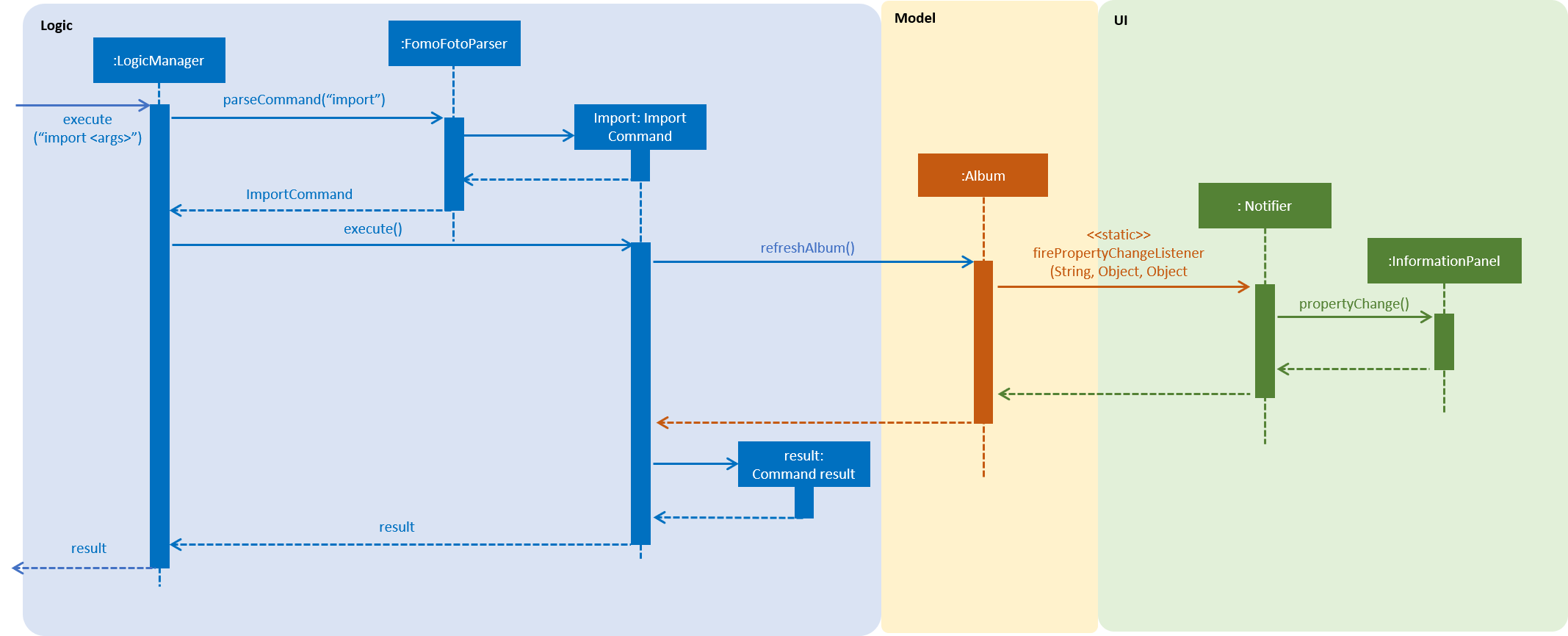
Design Considerations
-
Alternative 1: Import single images only.
-
Pros: Easy to implement.
-
Cons: Importing multiple images from the same directory needs repeated commands.
-
-
Alternative 2 (Current Choice): Import images from a directory as well.
-
Pros: Can import many images without repeating the command.
-
Cons: Can result in errors if too many images are imported or images are of alternative formats.
-
Assets and Temp Folder
The assets
and temp
directory from the storage component of FomoFoto. Both directories are created during runtime of the application.
The assets
directory is created in the same directory where FomoFoto is first launched and persist between sessions. The assets
directory is marked as FomoFoto.assets
.
The temp
directory is created per FomoFoto session. The target directory for the Temporary File
directory is in both Windows and OS X. The temp
directory is removed on program exit.
If FomoFoto is not given the permissions to write assets and temp to their respective directories i.e. limited write permissions, the application might not function properly.
|